
Start Writing Unit Test With Visual Studio For Mac
Shortcut keys for running tests in Visual Studio Posted by Peter Wibeck on Tuesday, February 18, 2014 Leave a Comment One of my most used shortcuts in Visual Studio is CTRL+R,T to debug the current test method from the code and CTRL+R,A to execute all tests.
This document describes how to create unit tests for your Xamarin.iOS projects.Unit testing with Xamarin.iOS is done using the Touch.Unit framework that includesboth an iOS test runner as well as a modified version of NUnit called Touch.Unit thatprovides a familiar set of APIs for writing unit tests.
Setting up a test project in Visual Studio for Mac
To setup a unit testing framework for your project, all you need to do is toadd to your solution a project of type iOS Unit Tests Project. Do thisby right-clicking on your solution and selecting Add > Add New Project. From thelist select iOS > Tests > Unified API > iOS Unit Tests Project(you can choose either C# or F#).
The above will create a basic project that contains a basic runner programand which references the new MonoTouch.NUnitLite assembly, your project willlook like this:
The AppDelegate.cs
class contains the test runner, and it looks likethis:
Note
The iOS Unit Test project type is not available in Visual Studio 2019 or Visual Studio 2017 on Windows.
Writing Some Tests
Now that you have the basic shell in place, you should write your first setof tests.
Tests are written by creating classes that have the [TestFixture]
attributeapplied to them. Inside each TestFixture class you should apply the [Test]
attribute to every method that you want the test runner to invoke. The actualtest fixtures can live in any file in your Tests project.
To quickly get started select Add/Add New File and select in the Xamarin.iOSgroup UnitTests. This will add a skeleton file that contains one passing test,one failing test and one ignored tests, it looks like this:
Running Your Tests
To run this project inside your solution right click on it and select Debug Itemor Run Item.
The test runner allows you to see which tests are registered and selectindividually which tests can be executed.
You can run individual test fixtures by selecting the text fixture from thenested views, or you can run all of your tests with 'Run Everything'. If you runthe default test that is supposed to include one passing test, one failure andone ignored test. This is what the report looks like, and you can drill downdirectly to the failing tests and find out more information about thefailure:
You can also look at the Application Output window in your IDE to seewhich tests are being executed and their current status.
Writing New Tests
NUnitLite is a modified version of the NUnit called Touch.Unitproject. It is a lightweight testing framework for .NET, based on theideas in NUnit and providing a subset of its features.It uses minimal resources and will run on resource-restricted platforms such asthose used in embedded and mobile development. The NUnitLite API is available to you inXamarin.iOS. With the basic skeleton provided by the unit test template, yourmain entry point are the Assert class methods.
In addition to the assert class methods, the unit testing functionality issplit on the following namespaces that are part of NUnitLite:
-->Use Visual Studio to define and run unit tests to maintain code health, ensure code coverage, and find errors and faults before your customers do. Run your unit tests frequently to make sure your code is working properly.
Create unit tests
This section describes how to create a unit test project.
IBM 4610 TF6 PRINTER DRIVER FOR MAC DOWNLOAD. Taiwanese Class A 46100 Statement These quiet, ibm 4610 tf6 thermal receipt printers deliver the same speed as the popular dual-station models: They are targeted to image-conscious retailers who require quiet printing at the front end of the store and need faster transaction times at the POS register. A00-1330,OVERVIEW Four new IBM SureMark Printer models will help retailers to attract and retain customers and streamline their operations. Now, both retail and food service environments can enjoy the benefits of the IBM SureMark printers. The TF6 is desi. Ibm 4610 tf6 printer driver for mac download.
Open the project that you want to test in Visual Studio.
For the purposes of demonstrating an example unit test, this article tests a simple 'Hello World' project named HelloWorldCore. The sample code for such a project is as follows:
In Solution Explorer, select the solution node. Then, from the top menu bar, select File > Add > New Project.
In the new project dialog box, find a unit test project template for the test framework you want to use and select it.
Click Next, choose a name for the test project, and then click Create.
Choose a name for the test project, and then click OK.
The project is added to your solution.
In the unit test project, add a reference to the project you want to test by right-clicking on References or Dependencies and then choosing Add Reference.
Select the project that contains the code you'll test and click OK.
Add code to the unit test method.
For example, for an MSTest project, you might use the following code.
Or, for an NUnit project, you might use the following code.
Tip
For more details about creating unit tests, see Create and run unit tests for managed code.
Run unit tests
Open Test Explorer.
To open Test Explorer, choose Test > Test Explorer from the top menu bar.
To open Test Explorer, choose Test > Windows > Test Explorer from the top menu bar.
Run your unit tests by clicking Run All.
After the tests have completed, a green check mark indicates that a test passed. A red 'x' icon indicates that a test failed.
Tip
You can use Test Explorer to run unit tests from the built-in test framework (MSTest) or from third-party test frameworks. You can group tests into categories, filter the test list, and create, save, and run playlists of tests. You can also debug tests and analyze test performance and code coverage.
View live unit test results
If you are using the MSTest, xUnit, or NUnit testing framework in Visual Studio 2017 or later, you can see live results of your unit tests.
Note
Live unit testing is available in Enterprise edition only.
Turn live unit testing from the Test menu by choosing Test > Live Unit Testing > Start.
View the results of the tests within the code editor window as you write and edit code.
Click a test result indicator to see more information, such as the names of the tests that cover that method.
For more information about live unit testing, see Live unit testing.
Generate unit tests with IntelliTest
When you run IntelliTest, you can see which tests are failing and add any necessary code to fix them. You can select which of the generated tests to save into a test project to provide a regression suite. As you change your code, rerun IntelliTest to keep the generated tests in sync with your code changes. To learn how, see Generate unit tests for your code with IntelliTest.
Tip
IntelliTest is only available for managed code that targets the .NET Framework.
Analyze code coverage
To determine what proportion of your project's code is actually being tested by coded tests such as unit tests, you can use the code coverage feature of Visual Studio. To guard effectively against bugs, your tests should exercise a large proportion of your code. To learn how, see Use code coverage to determine how much code is being tested.
Use a third-party test framework
You can run unit tests in Visual Studio by using third-party test frameworks such as Boost, Google, and NUnit. Use the NuGet Package Manager to install the NuGet package for the framework of your choice. Or, for the NUnit and xUnit test frameworks, Visual Studio includes preconfigured test project templates that include the necessary NuGet packages.
To create unit tests that use NUnit:
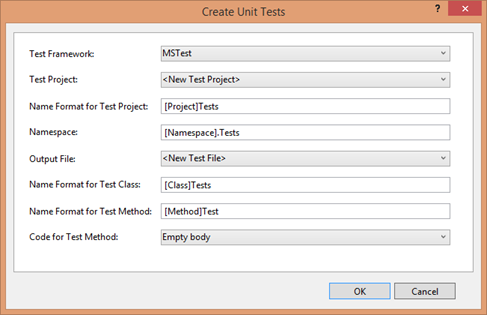
Open the solution that contains the code you want to test.
Right-click on the solution in Solution Explorer and choose Add > New Project.
Register for the Client In order to use Badlion Client, you are going to need to have an account in the forums. If you already have an account, then lucky you all you have to do is log into it and you can skip this step. Badlion Client has pulled through once again! This update brings Mac Players onto Badlion and Badlion Client is now open to not just Windows, but Mac players now too! This is a great update. How to download Badlion Client on Mac has based on open source technologies, our tool is secure and safe to use. This tool is made with proxy and VPN support, it will not leak your IP address, 100% anonymity, We can't guarantee that.
Don’t forget to read instructions after installation. Enjoy How to install badlion client (Can’t on Mac OS). For MAC OS/X. All files are uploaded by users like you, we can’t guarantee that How to install badlion client (Can’t on Mac OS) For mac are up to date. The Badlion Network is an online gaming network that provides competitive PvP for Minecraft with ArenaPvP, UHC, Survival Games. Play for free today!
Select the NUnit Test Project project template.
Click Next, name the project, and then click Create.
The project template includes NuGet references to NUnit and NUnit3TestAdapter.
Add a reference from the test project to the project that contains the code you want to test.
Right-click on the project in Solution Explorer, and then select Add > Reference. (You can also add a reference from the right-click menu of the References or Dependencies node.)
Add code to your test method.
Run the test from Test Explorer or by right-clicking on the test code and choosing Run Test(s).